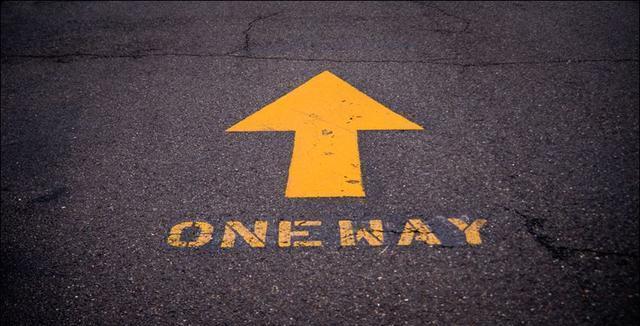
经典实现的手写
Function.prototype.bind
1 | // 定义 |
防抖
1 | const debounce = function(cb,timeout = 500,immediater = false){ |
节流
1 | const throttle = function(cb,timeout = 500,immediate = true){ |
本文是原创文章,采用CC BY-NC-SA 4.0协议,完整转载请注明来自黑凤梨的博客
评论
1 | // 定义 |
1 | const debounce = function(cb,timeout = 500,immediater = false){ |
1 | const throttle = function(cb,timeout = 500,immediate = true){ |